Building a monitoring solution for Office 365 service availability using Azure Logic Apps
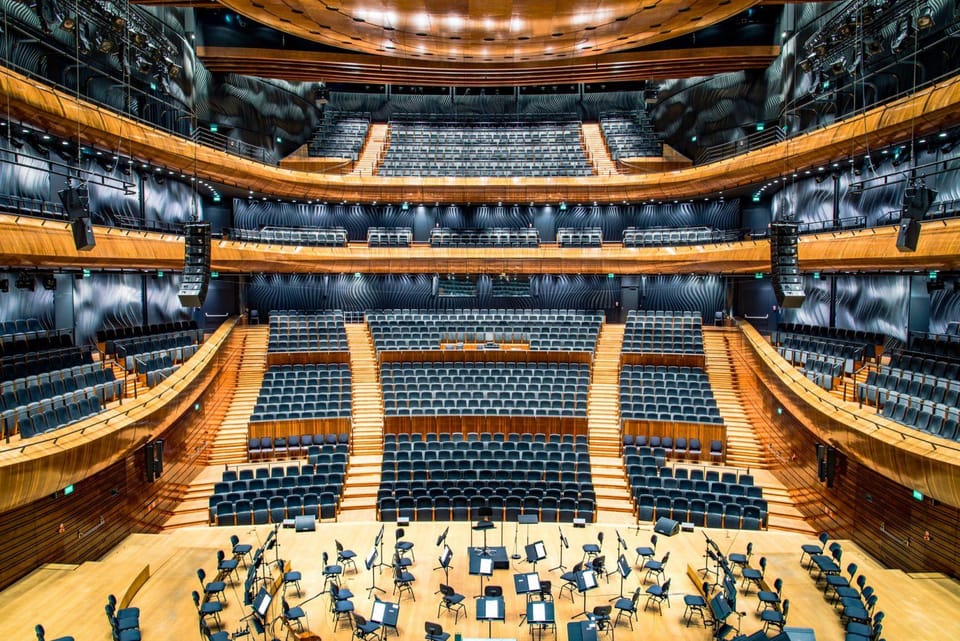
I spent some time in early December building an integration between Azure Log Analytics (and Azure Sentinel, obviously) and Office 365 Audit Logs. The latter exposes the logs through Office 365 Management Activity API, which is very handy, albeit slightly cumbersome to use. I wrote about that experience here.
I noticed that there is also a separate Office 365 API I found useful, called the Office 365 Service Communications API. It’s much simpler to use, and exposes a limited set of data – Get Services, Get Current Status (of services), Get Historical Status, Get Messages and show Errors. Using this data, it’s easy to monitor what the status of your Office 365 tenant is, and if any of the services are degraded.
Getting started: Creating an Azure AD App
First, I need to provision an app in Azure Active Directory. This is so that I can then authenticate via Azure AD, and gain access to whatever services I need through OAuth2.
Navigate to Azure Portal, and then select Azure AD. From here, select App registrations. Click New registration. Fill in the name, and leave other selections as defaults.
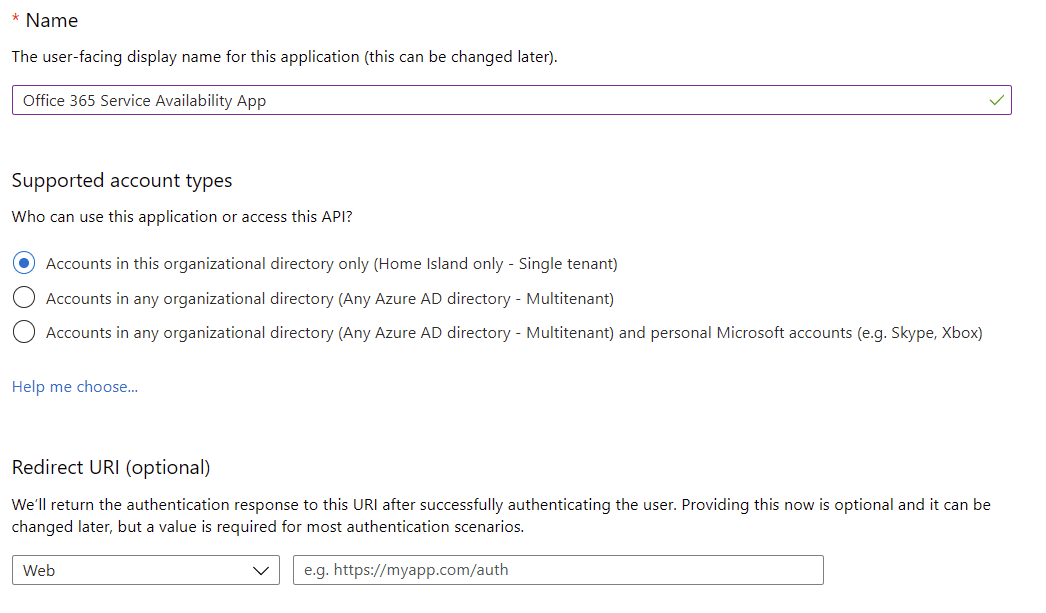
Once you click OK, you have an Azure AD-supported app! We need to grant permissions next so that we can actually leverage the Office 365 Management APIs. Under the app, select API Permissions.
The permission you need to grant for the app, under API Permissions, is ServiceHealth.Read for the Office 365 Management APIs. Click Add a permission, and select Office 365 Management APIs:

Then, select Application permissions, as we’ll run this solution in the background without a signed-in user. Under Select permissions, choose ServiceHealth.Read:
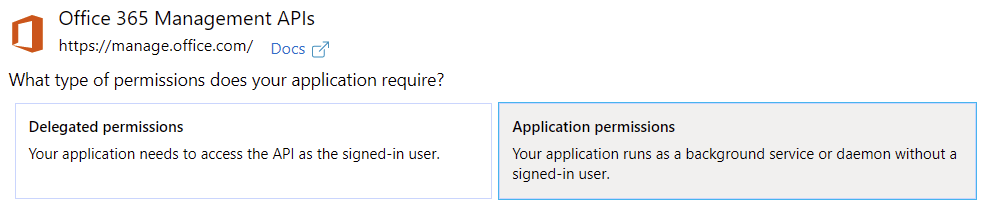
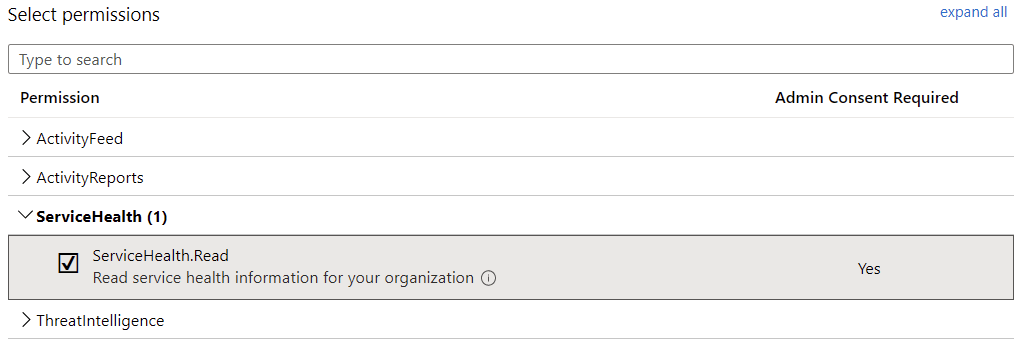
You’ll also want to grant admin consent next, but first, you have to wait for a few minutes for the permissions to kick in.

Click Grant admin consent on TENANT, when the button becomes available. You’ll need to authenticate once more to perform this task.
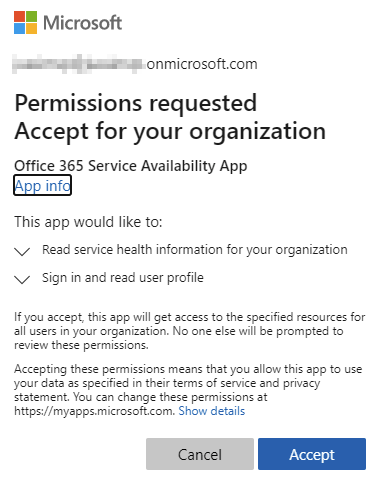
Once completed successfully, you should have granted admin consent successfully, and you’ll see a green checkmark:

Finally, generate the client secret under Certificates & secrets, by clicking on New client secret. Usually, it makes sense not to set expiration to Never, so perhaps choose 1 or 2 years, and click Add.
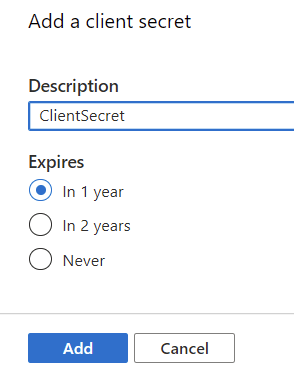
Record the value of the client secret, as you’ll only see it once here:
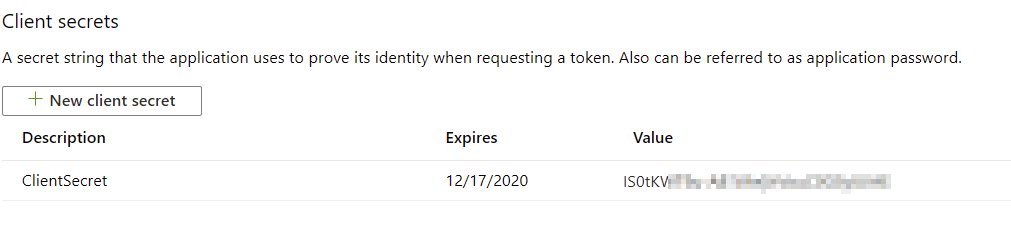
And that’s it! You should now have an Azure AD-supported app, with permissions to query the Service Health of your Office 365 tenant.
Building a Proof of Concept using PowerShell
I built a 5-minute Proof of Concept hack to try, and get data from the API. It worked, much to my surprise.
As before, I needed to define a few variables to hold values – namely, the OAuth2-based authorization data. So first, define the following:
$clientID = "CLIENT_ID"
$clientSecret = "CLIENT_SECRET"
$loginURL = "https://login.microsoftonline.com/"
$resource = "https://manage.office.com"
$tenantID = "TENANT_ID"
$body = @{grant_type="client_credentials";resource=$resource;client_id=$clientID;client_secret=$clientSecret}
$oauth = Invoke-RestMethod -Method Post -Uri $loginURL/$tenantID/oauth2/token?api-version=1.0 -Body $body
$headerParams = @{'Authorization'="$($oauth.token_type) $($oauth.access_token)"}
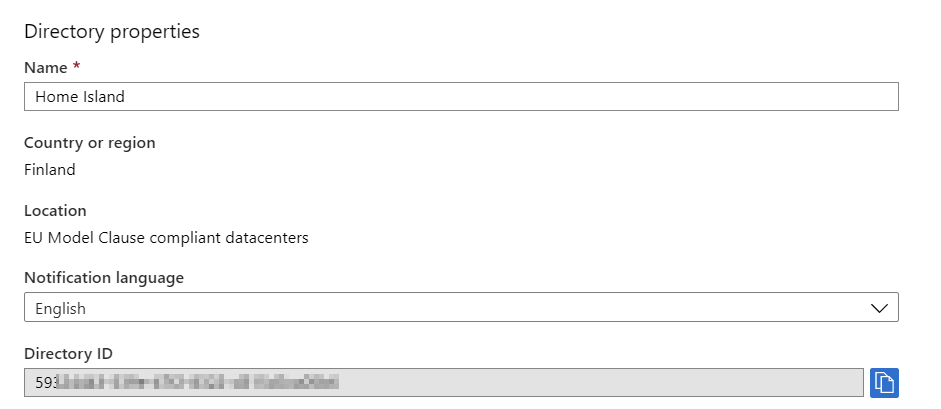
az account show
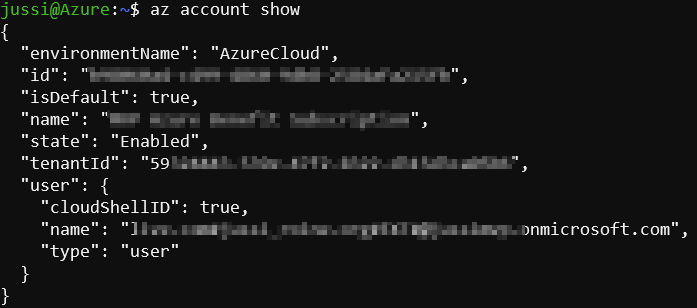